Firebase Web documentSnapshots vs querySnapshots
How to handle querySnapshots and documentSnapshots in your Firebase web project.
When I first started developing with Firebase one concept that confused the heck out of me as simple as it sounds was the different between a documentSnapshot and a querySnapshot. So let's dive in and talk about the difference's and how to use them in a Web based HTML project.
If you're familiar with the concept of document based databases which you should be if you are programming with Firebase. You'll know that you have groups of documents which are called collections.
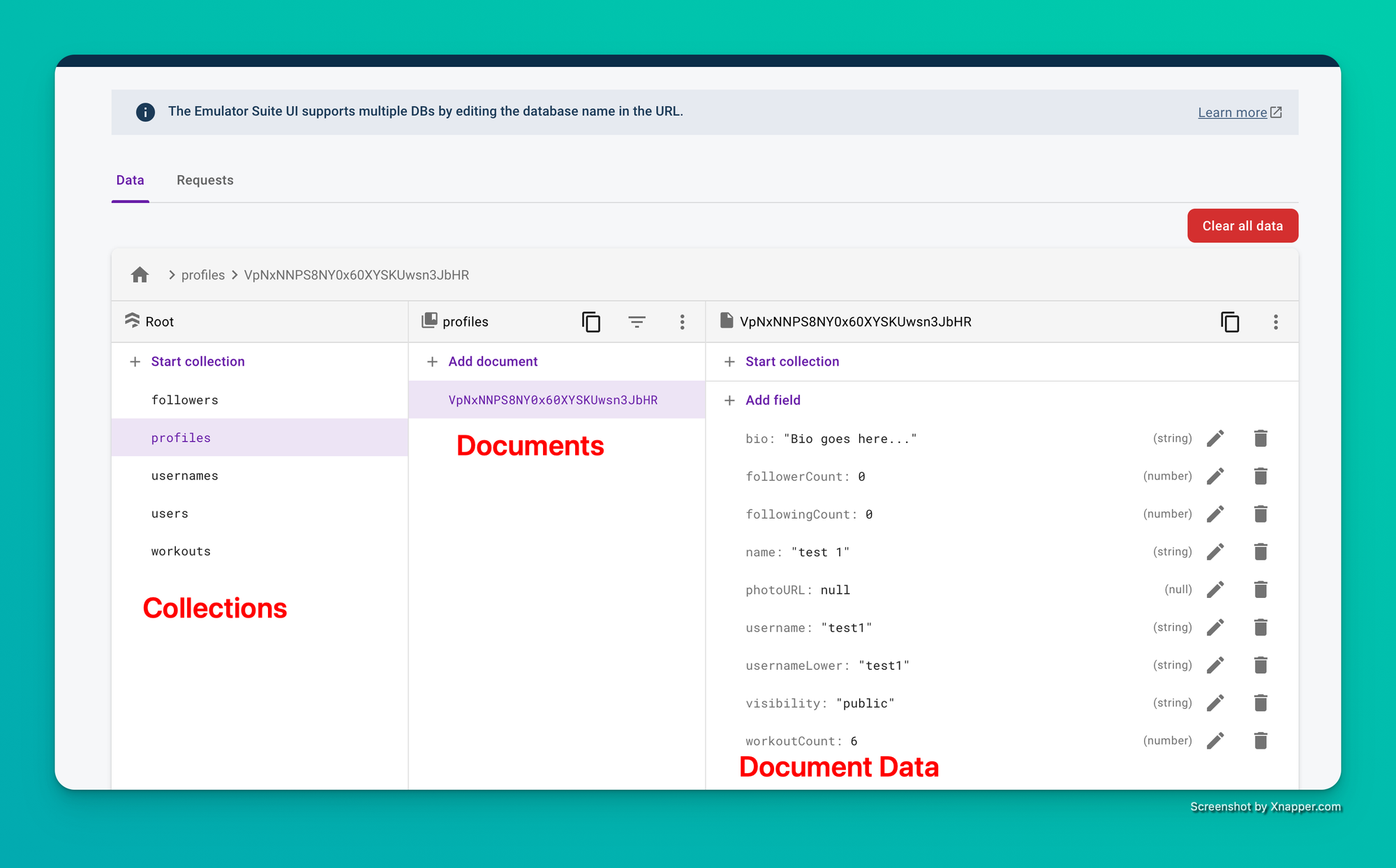
Knowing how this works makes figuring out the difference between a documentSnapshot and a querySnapshot very easy.
Collections are querySnapshots
Documents are documentSnapshots (Duh)
Okay simple enough right, from this then you'll know a querySnapshot is a collection of documents based on the query that you used to return that data.
export const randomFunctionName = () => {
const constraints = [
where('user.id', '==', user),
]
const collectionRef = collection(
firestore,
workoutCollection // example
) as CollectionReference
const dbQuery = query(collectionRef, ...constraints)
return getDocs(dbQuery)
}
Above is an example of how it might look in your code when you return a querySnapshot in your code. Using the function getDocs returns a promise which on a ReactJs application might look like.
randomFunctionName()
.then((querySnapshot) => {
if (querySnapshot.empty) {
return
}
})
Here you'll notice I've set the .then callback value to be querySnapshot which makes it so much easier to understand. From here you'll know ahh okay this function is returning a querySnapshot which means I can call querySnapshot.empty to check if I have any documents returned from this query call (Remember a query is basically checking for documents inside a collection).
From here of course you can just return a singular document rather than a collection of documents in a querySnapshot call.
export const randomFunctionName2 = () => {
return getDoc(doc(firestore, 'users', user))
}
This is how your call might look in firebase to return a documentSnapshot which is what's returned when using getDoc. Remember this is also a promise value so you need to make sure you handle it accordingly.
randomFunctionName2().then((documentSnapshot) => {
if (!documentSnapshot.exists()) {
return
}
})
So here you can see to actually check if there is data inside a documentSnapshot you use the documentSnapshot.exists() function that will return true or false depending on if that document was actually returned.
Conclusion
Now you've learned the difference between querySnapshots and documentSnapshots and how you would go about handling them in your Web JavaScript based project. As you've seen the main difference between handling them is either using .exists() or .empty depending on if you use getDoc or getDocs.
Hopefully this helped, happy developing!